Hello World – micro:bit MicroPython with Mu
In this project you’ll learn how to create your first program in MicroPython, and how to download and run it on a micro:bit.
You’ll need:
- A computer with the Mu editor, which you can download here: https://codewith.mu/
- A micro:bit
- A USB cable to link your micro:bit to the computer
You’ll learn
- How to code in Python using the Mu editor
- How to check your code
- How to flash your code onto a micro:bit
- How to display text on a micro:bit
- How to display an image on a micro:bit
- How to comment your code
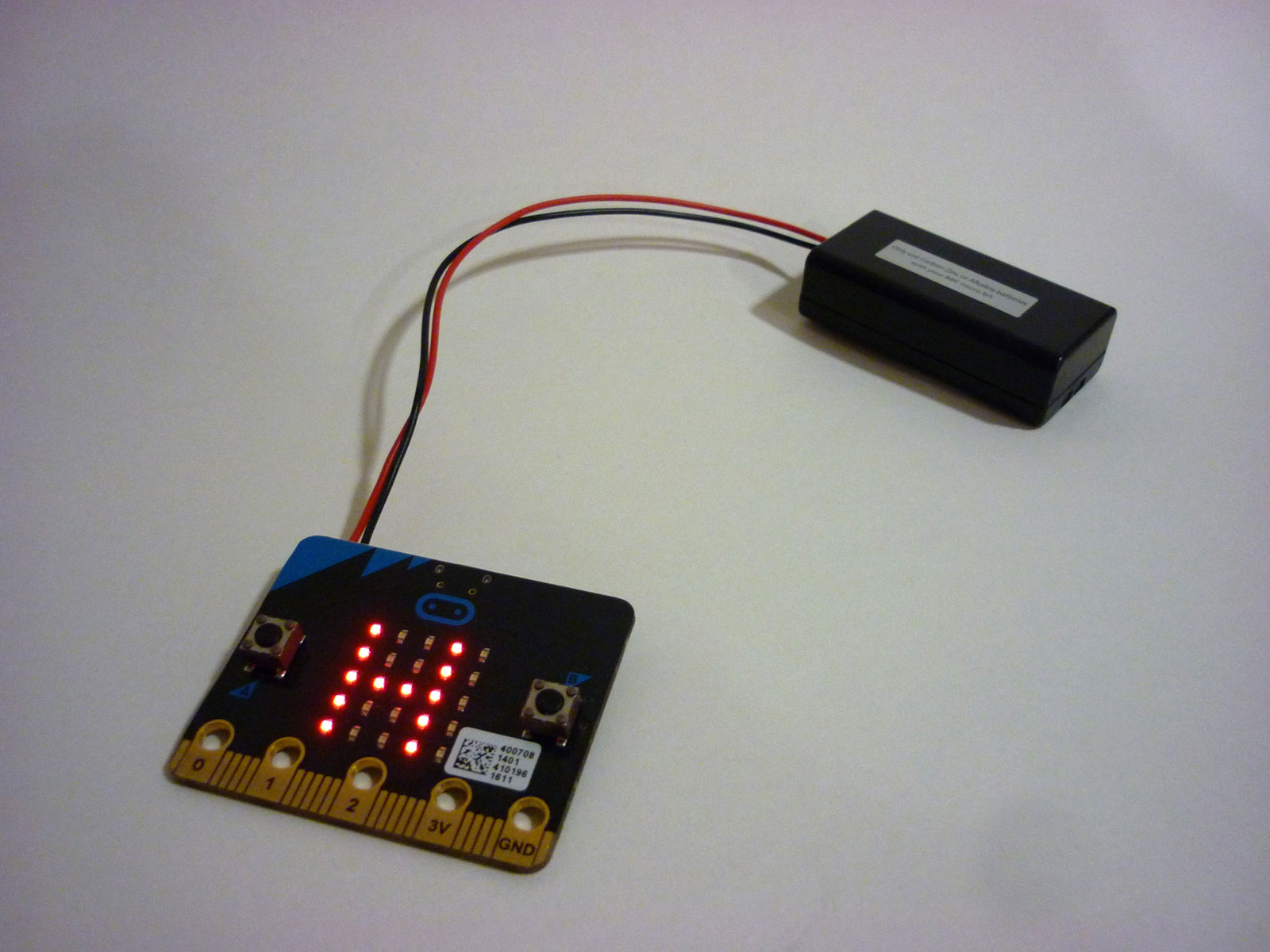
1. The Mu editor
On your computer desktop, look for the Mu icon, which looks like this:
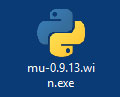
Double-click to open Mu and you’ll see the editor:
You might see the last code that was created. To clear this, click on the New button.
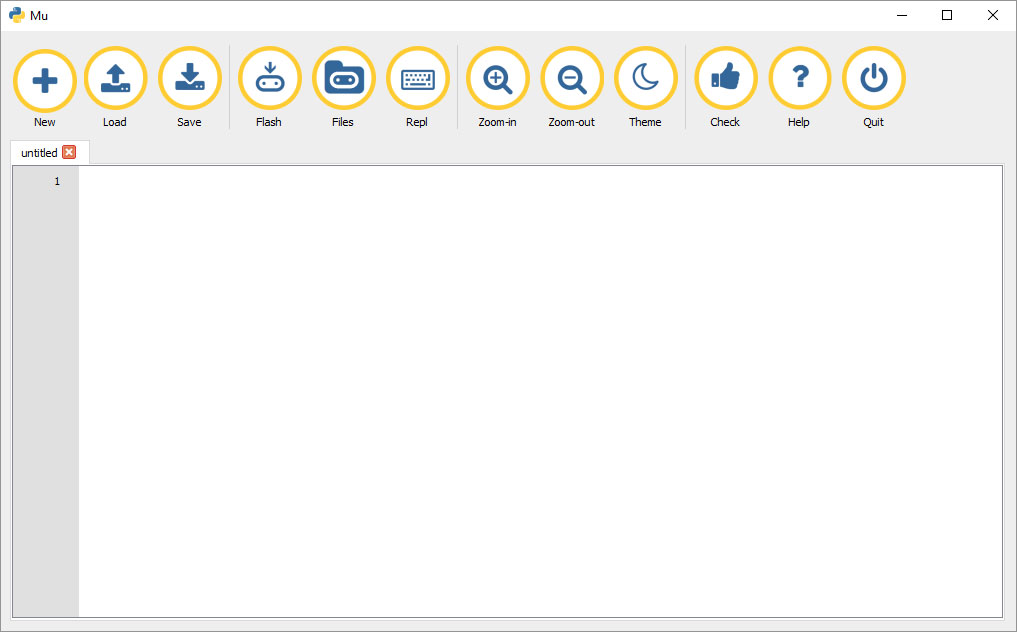

Clears any code in the editor so that you can start a new program.

Flashes the program to the micro:bit.

Loads a program that you have previously saved.

Checks your code for errors.

Save the program to your computer.
2. Hello World using MicroPython
When programmers are learning a new language, we tend to write a short line program that prints the message “Hello world!”. Type in the code below, one line at a time and read the notes underneath to what each line does.
Make sure your code is indented exactly as above so that all three lines are repeated so that after the sleep command the program will go back to the display.scroll command.
Before we flash this onto the micro:bit we need to save it. Click on the Save button and save to a folder on your computer or network. Name the file hello world. Once it’s save you’ll notice that Mu adds the correct file extension so it becomes hello world.py.
# Hello World by Richard
from microbit import *
while True:
display.scroll('Hello World!')
display.show(Image.HEART)
sleep(2000)
# Hello World by Richard
Any line that starts with a # is a comment. Use these to add notes to your code so you know what it’s doing when you look at it later. Change the comment to show your name.
from microbit import *
Python needs some special code to work with micro:bits. Someone has already written this code and all you have to do is import their library, called microbit. So this line means: from the microbit library import all the code (* means all).
while True:
This is a loop. All the lines of code that appear in the block of code underneath will be repeated while the thing we are checking is true. In this case the thing we are checking is True, which is always true so the loop will continue forever!
Note how the following code after the while is indented to the right. This is important as it shows which lines need to be repeated each time we go around the loop.
display.scroll('Hello World!')
display refers to the 25 red LEDs on the micro:bit so this will scroll the text in the brackets across the LEDs.
display.show(Image.HEART)
This will show an image of a heart on the display.
sleep(2000)
The micro:bit will sleep for 2,000 milliseconds. That’s the same as 2 seconds.