Beethoven – making music with micro:bit and MicroPython
In this project we get the micro:bit to play some music, including (a bit of) Beethoven’s 5th Symphony.
You’ll need:
- A micro:bit
- A USB cable to link your micro:bit to the computer
- A battery pack
- Two crocodile clip connectors
- A speaker or headphones with a standard 3.5mm jack
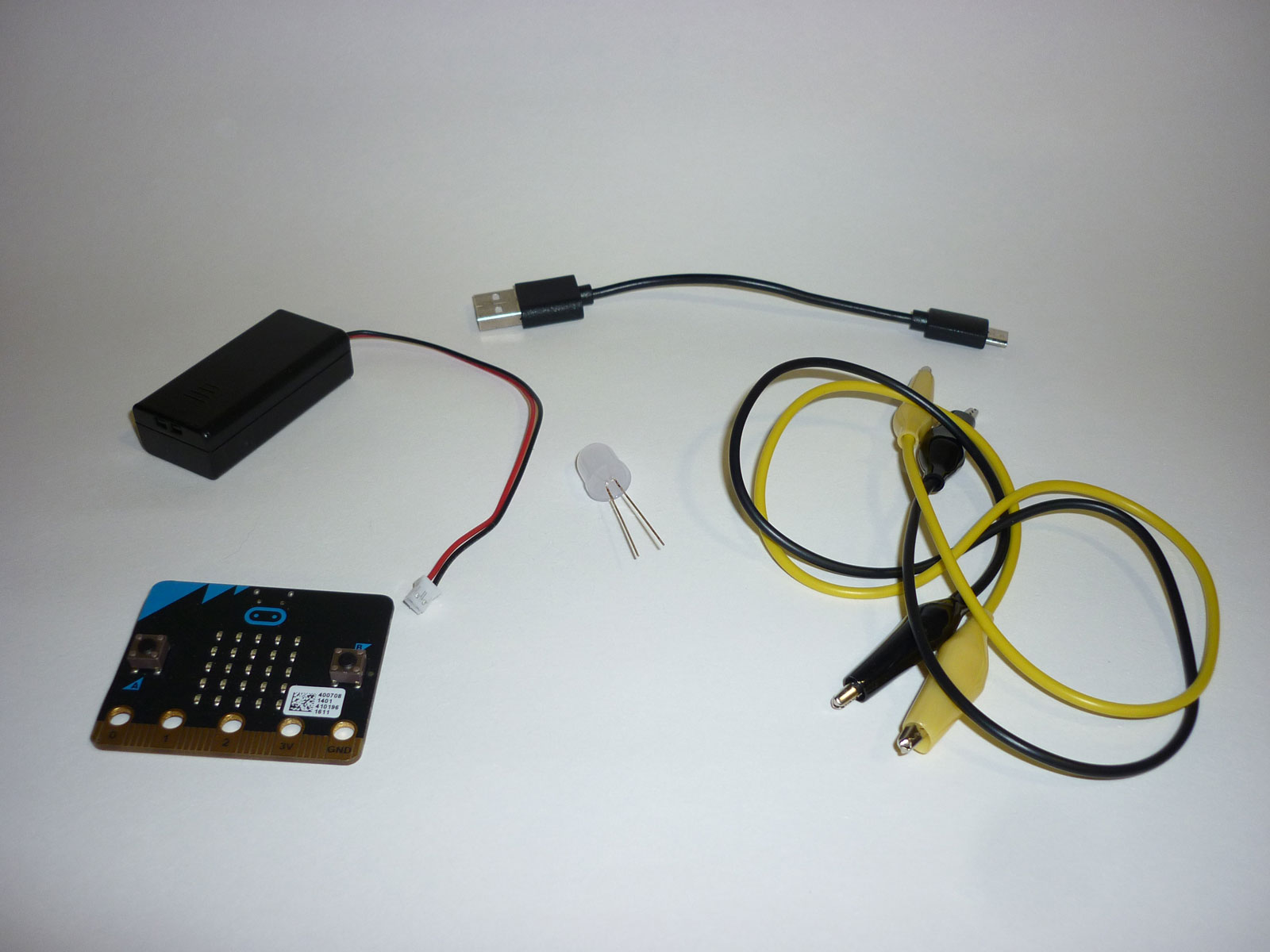
-
Connect your micro:bit
Using your crocodile clips, carefully connect:
- the Ground (GND) pin to the base of the speaker cable jack
- Data pin 0 (0) to the end of the jack
It should look like this:
Make sure that the clips on the speaker jack only touch the top and bottom sections, and don’t touch each other.
Load the Mu editor and type in the code to the right:
First we need to load the two micro:bit libraries so that we can access the microbit and music functions
You play music using the music.play function. There are some tunes built into the micro:bit which you use as here:
# Load the micro:bit libraries that we need
import microbit
import music
music.play(music.PYTHON)
To play a single note, you use the note itself (a, b, c, d, e, f. g) in single quotes.
You can play a tune using a list of notes. A list is a collection of values and is enclosed by square brackets [] with each value separated by a comma.
Using a variable for the tune makes this a bit neater. Here we’ve used a variable called scale.
# Load the micro:bit libraries that we need
import microbit
import music
scale = ['a','b','c','d','e','f','g']
music.play(scale)
We could create a number of tunes and play them when buttons are pressed, or perhaps randomly.
Our complete code uses the buttons to play some Beethoven or the Python tune.
# Add your Python code here. E.g.
import microbit
import music
a = ['c1:4', 'e:2', 'g', 'c2:4']
b = ['r4:2', 'g', 'g', 'g', 'eb:8',
'r:2', 'f', 'f', 'f', 'd:8']
music.set_tempo(bpm=180)
while True:
if microbit.button_a.is_pressed():
music.play(a)
elif microbit.button_b.is_pressed():
music.play(b)
More Music
You’ll see some extra bits in the notes in the Beethoven tune. Here are some extra things you can do to change notes and tunes:
Octaves
‘c1’ is from octave 1, ‘c4’ is from octave 4. Try these in a tune and see how they sound different.
Ticks
Ticks let you change the length of each note. A note will be 4 ticks long unless you change this.
‘a:2’ is note A lasting 2 ticks – half the length
‘d2:8’ is note D in octave 2, lasting 8 ticks, twice as long
Rests
‘r’ is a rest (of 4 ticks)
‘r:8’ is a 2 note rest
More Tunes
Here are some more built-in tunes to try:
- DADADADUM – the opening to Beethoven’s 5th Symphony in C minor.
- ENTERTAINER – the opening fragment of Scott Joplin’s Ragtime classic “The Entertainer”.
- PRELUDE – the opening of the first Prelude in C Major of J.S.Bach’s 48 Preludes and Fugues.
- ODE – the “Ode to Joy” theme from Beethoven’s 9th Symphony in D minor.
- NYAN – the Nyan Cat theme (http://www.nyan.cat/). The composer is unknown. This is fair use for educational porpoises (as they say in New York).
- RINGTONE – something that sounds like a mobile phone ringtone. To be used to indicate an incoming message.
- FUNK – a funky bass line for secret agents and criminal masterminds.
- BLUES – a boogie-woogie 12-bar blues walking bass.
- BIRTHDAY – “Happy Birthday to You…” for copyright status see: http://www.bbc.co.uk/news/world-us-canada-34332853
- WEDDING – the bridal chorus from Wagner’s opera “Lohengrin”.
- FUNERAL – the “funeral march” otherwise known as Frédéric Chopin’s Piano Sonata No. 2 in B♭ minor, Op. 35.
- PUNCHLINE – a fun fragment that signifies a joke has been made.
- PYTHON – John Philip Sousa’s march “Liberty Bell” aka, the theme for “Monty Python’s Flying Circus” (after which the Python programming language is named).
- BADDY – silent movie era entrance of a baddy.
- CHASE – silent movie era chase scene.
- BA_DING – a short signal to indicate something has happened.
- WAWAWAWAA – a very sad trombone.
- JUMP_UP – for use in a game, indicating upward movement.
- JUMP_DOWN – for use in a game, indicating downward movement.
- POWER_UP – a fanfare to indicate an achievement unlocked.
- POWER_DOWN – a sad fanfare to indicate an achievement lost.
Soil Moisture Sensor for micro:bit
micro:bit, micro:bit Connecting, Moisture